By: F09-B1
Since: Jun 2016
Licence: MIT
- 1. Introduction
- 2. How to Contribute to Your TA
- 3. Setting Up
- 4. Design
- 5. Implementation
- 6. Documentation
- 7. Testing
- 8. Dev Ops
- Appendix A: Product Scope and Features
- Appendix B: User Stories
- Appendix C: Use Cases
- Appendix D: Non Functional Requirements
- Appendix E: Glossary
- Appendix F: Instructions for Manual Testing
1. Introduction
Your TA is an address book application designed to enhance the capabilities of a university Teaching Assistant (TA) or tutor.
It aims to provide all the essential functions needed to manage the administrative duties of handling a class as well as
to aid the tutor to better connect with the class.
It includes a calendar, scheduling capabilities, as well as the ability to mark attendance and participation.
2. How to Contribute to Your TA
2.1. New developers
You may wish to refer to Section 3: Setting up to properly set up the Your TA project file.
Read through the rest of the guide to familiarise yourself with how Your TA components functions together.
Try making some enhancements or edits to a component and make a pull request (PR) to our repo! We will take a look at your PR and give you some feedback or even merge your code if it’s a good working enhancement.
2.2. Experienced developers
The developer guide contains the design of the models that make up the program. Each section also details how each and every functionality
is implemented and how it interacts with the different components.
We would greatly appreciate your help in fine-tuning the components and also welcome any suggestions or feedback to improve Your TA.
3. Setting Up
This section contains the steps needed to properly set up the required files to work on the program source code and to ensure the application runs properly.
3.1. Prerequisites
The following are essential programs and libraries needed to start developing Your TA.
-
JDK
1.8.0_60
or laterHaving any Java 8 version is not enough.
This app will not work with earlier versions of Java 8. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them. -
Sufficient Java and Javafx knowledge
This application is written in Java and Javafx. A good amount of experience with the language (about 10k lines of code written in Java and Javafx) is required to understand and work in the application.
3.2. Setting up the project in your computer
Please follow the steps below to download and set up the project.
-
Fork this repository, and clone the fork to your computer.
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first). -
Set up the correct JDK version for Gradle.
-
Click
Configure
>Project Defaults
>Project Structure
. -
Click
New…
and find the directory of the JDK.
-
-
Click
Import Project
. -
Locate the
build.gradle
file and select it. ClickOK
. -
Click
Open as Project
. -
Click
OK
to accept the default settings. -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
3.3. Verifying the setup
Run the following steps to ensure that you successfully set up the project.
-
Run the
seedu.address.MainApp
and try a few commands. -
Run the tests to ensure they all pass.
You should see in the console that all the tests have been successfully completed.
3.4. Configurations to do before writing code
The following configurations should be set up before you start coding to ensure a uniform coding style.
3.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify:
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
3.4.2. Updating documentation to match your fork
After forking the repo, links in the documentation will still point to the CS2103JAN2018-F09-B1/main
repository. If you plan to develop this as a separate product (i.e. instead of contributing to the CS2103JAN2018-F09-B1/main
) , you should replace the URL in the variable repoURL
in DeveloperGuide.adoc
and UserGuide.adoc
with the URL of your fork.
3.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
3.4.4. Getting started with coding
When you are ready to start coding, you may:
-
Get some sense of the overall design by reading Section 4.1, “Architecture”.
-
Take a look at [GetStartedProgramming].
4. Design
This section will go through the design aspect of Your TA. It contains the architecture of the application and how the components that make up the program function and interact with one another in more detail.
4.1. Architecture
Figure 1 below is the architecture diagram explains the high-level design of the application. It shows all of the components of the application and how they interact with one another.
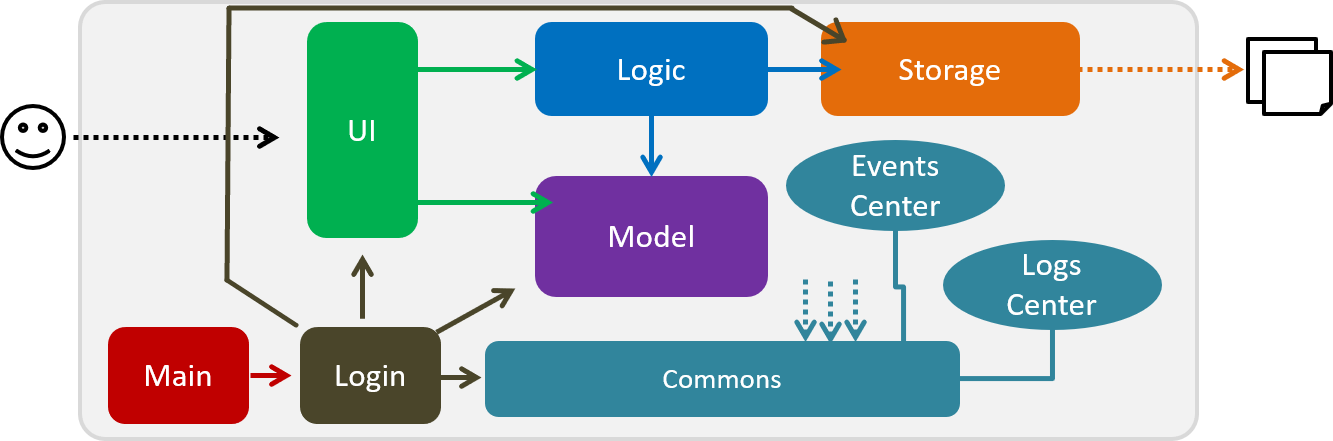
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Given below is a quick overview of each component.
Main
has only one class called MainApp
. It is responsible for:
-
Initializing the components in the correct sequence at the app launch, and connects them up with each other.
-
Shutting down the components and invokes cleanup method where necessary when the application exits.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
The classes can be found in the seedu.addressbook.commons
package.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design). -
LogsCenter
: Used by many classes to write log messages to the app’s log file.
The rest of the App consists of the following five components:
Do note that each of the components
-
Defines its API in an
interface
with the same name as the component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see Figure 2 given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
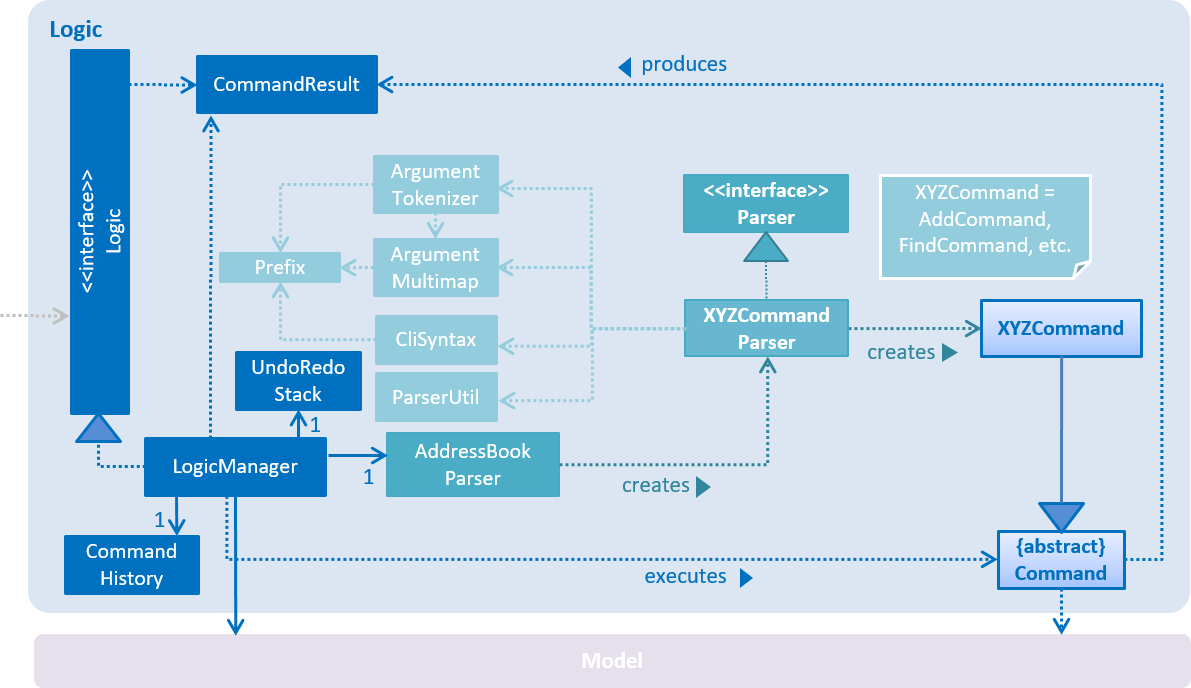
Events-Driven nature of the design
Your TA’s design is based on an event driven architecture. This allows the different components to communicate with another by utilizing events.
The Sequence Diagram (Figure 3) below shows how the components interact for the scenario where the user issues the command delete 1
.
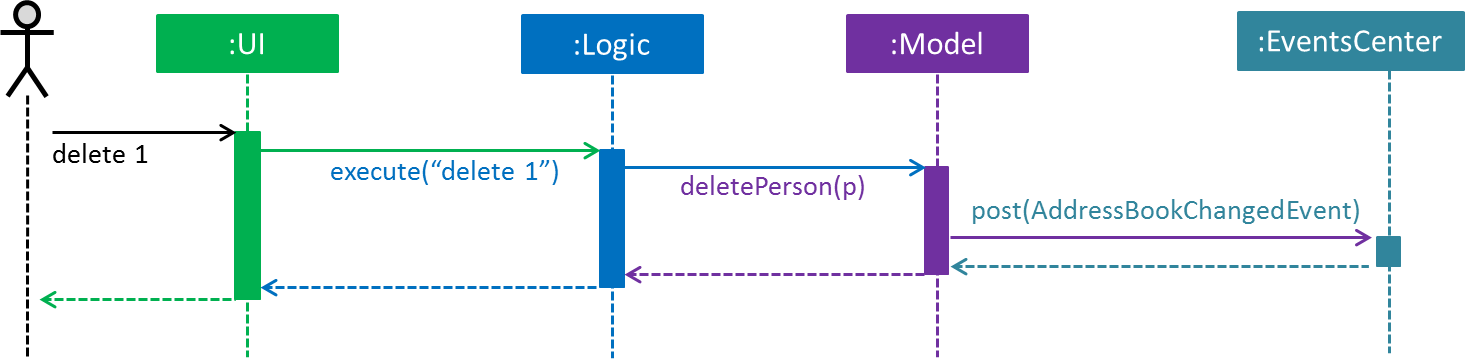
delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when Your TA data is changed, instead of asking the Storage to save the updates to the hard disk.
|
Figure 4 below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
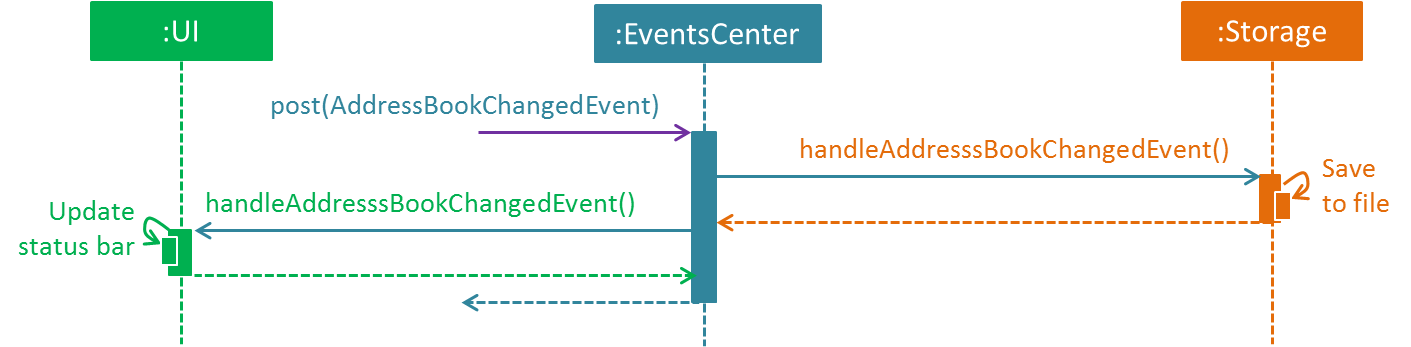
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
4.2. Login component
Before access to the application is granted, the user is required to log in with their Username
and Password
(see Figure 5).
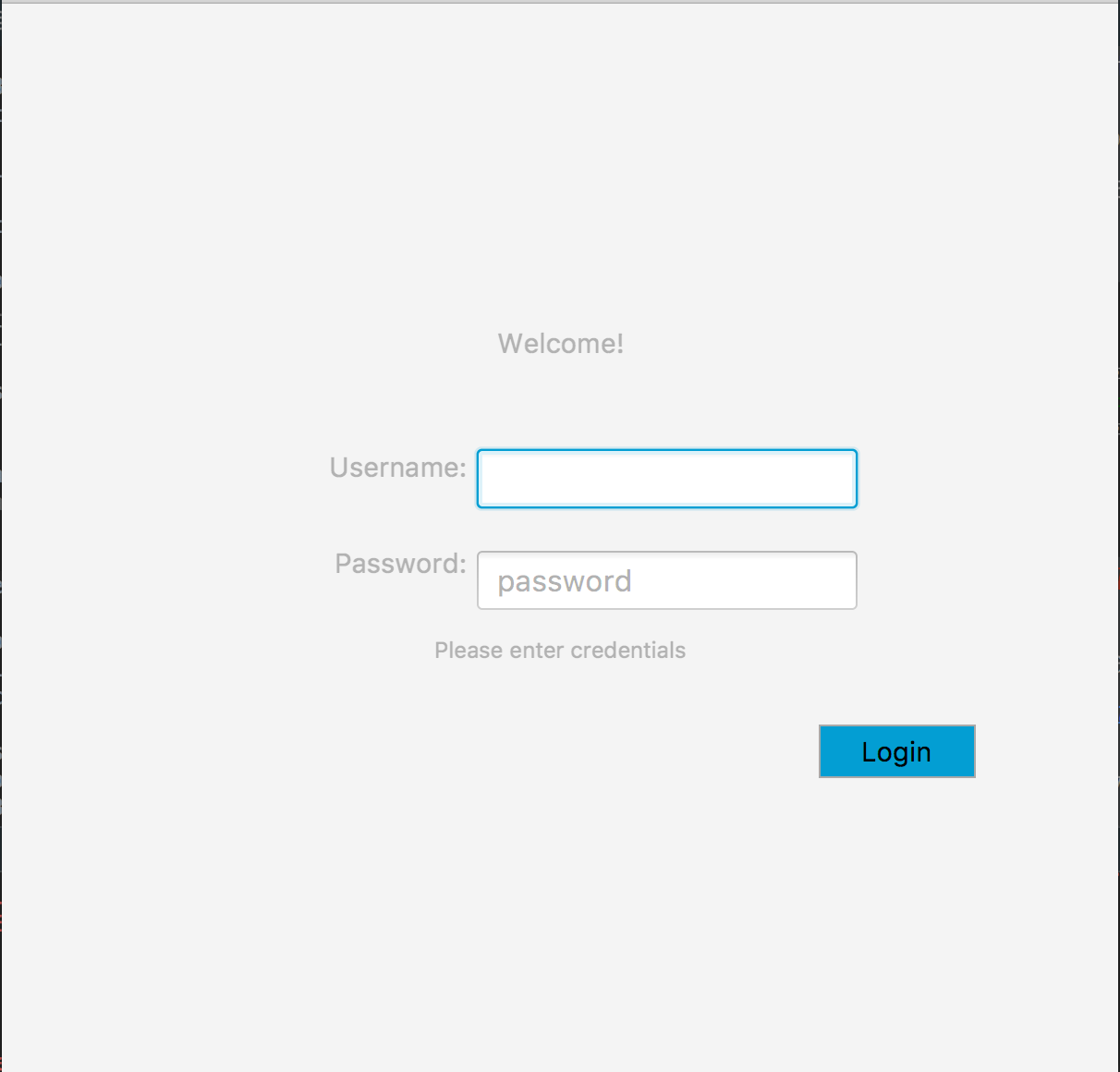
Upon launching the app, the Login component takes in two inputs from the user: Username
and Password
, creates an account, then stores the user’s login credentials into a .xml
file.
If that .xml
file already exists (Username
entered is existing Username
), it authenticates the User then loads in data previously saved by that User.
[Optional] .xml
file is encrypted.
-
The login credentials are therefore immutable (cannot be changed).
-
The same username and password have to be used every time the user wishes to access the app.
Username
andPassword
are case-sensitive. -
Only upon successful authentication will the app load data from the
Storage
Component.
4.3. UI component
The UI component handles to interaction between Your TA and the user. It itself contains other smaller components that build up to form the entire UI.
The figure below shows what makes up the structure of the UI component.
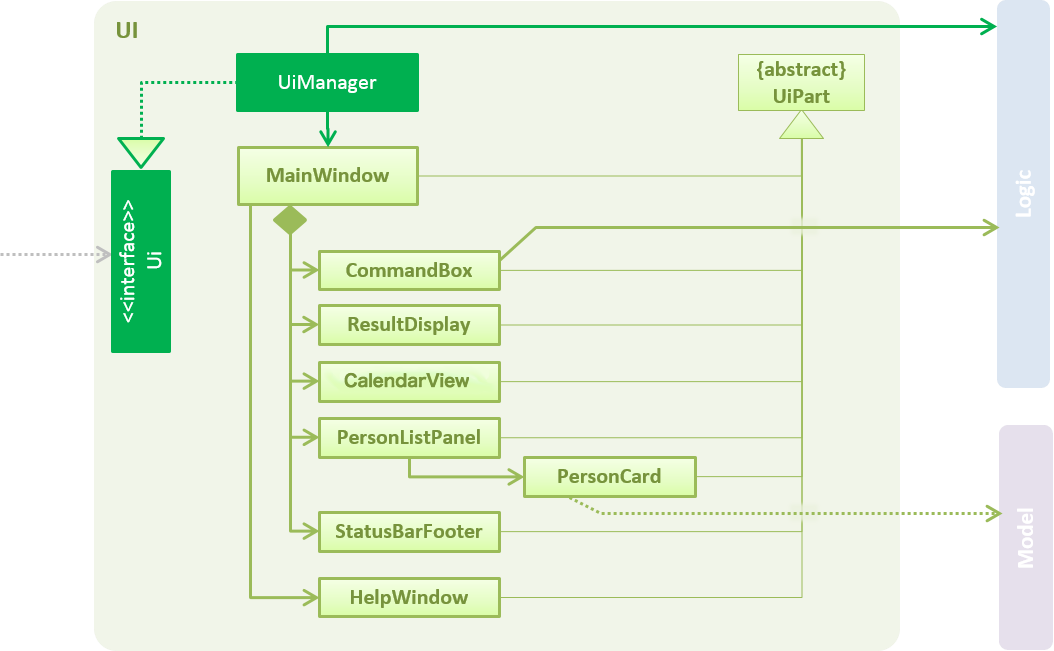
API : Ui.java
As seen in Figure 6, the UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, TodoListPanel
, StatusBarFooter
, CalendarView
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component loads the layout of each part from the .fxml
file and then does the bindings to various variables in the address book model in the corresponding .java
file.
It may be difficult to edit the .fxml file directly. Javafx Scene Builder is recommended to be used to edit or create new .fxml files.
|
The UI
component:
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
4.4. Logic component
The logic component controls how Your TA functions upon the different commands that it supports.
Figure 7 below shows how the LogicManager
functions in the application.
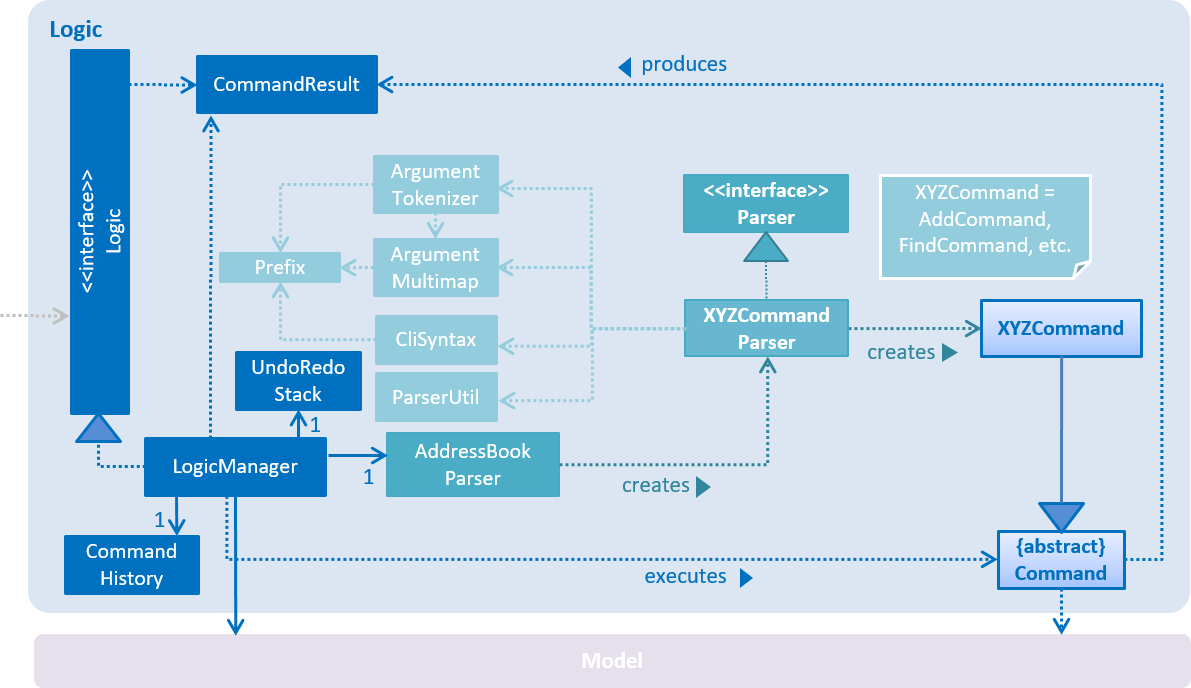
Figure 8 below shows finer details concerning XYZCommand
and Command
in Figure 7, “Structure of the Logic Component”.
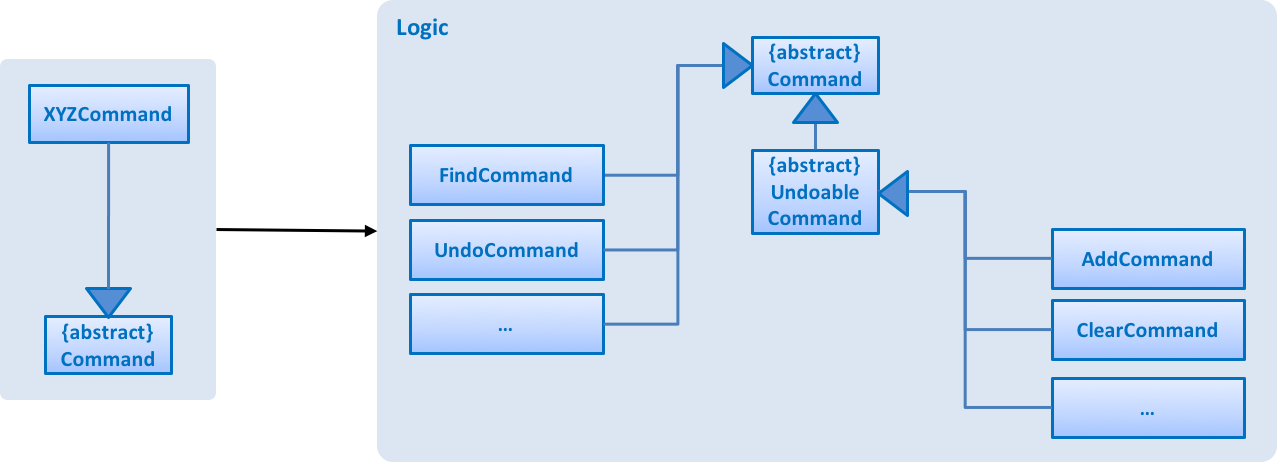
API :
Logic.java
The following steps are a brief overview of how a command is handled by the logic component.
-
Logic
uses theAddressBookParser
class to parse the user command. -
This results in the creation of a
Command
object which is executed by theLogicManager
. The command execution can affect theModel
(e.g. adding a person) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUI
. -
The
UI
then displays to the user the changes and the result of the command.
Figure 9 below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
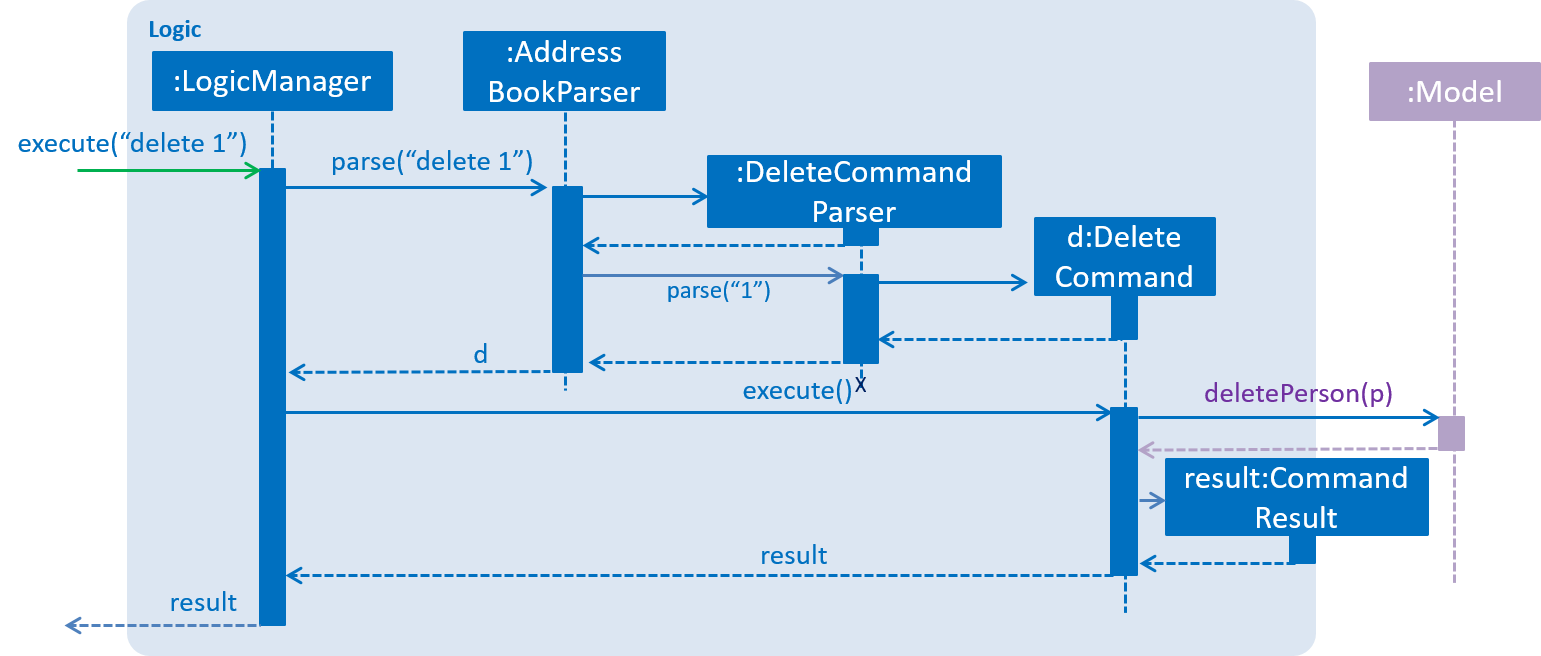
delete 1
Command4.5. Model component
The model component tackles how Your TA maintains the data it holds and how the data is communicated between each major component.
Figure 10 shows the different components and interfaces that make up the Model
component.
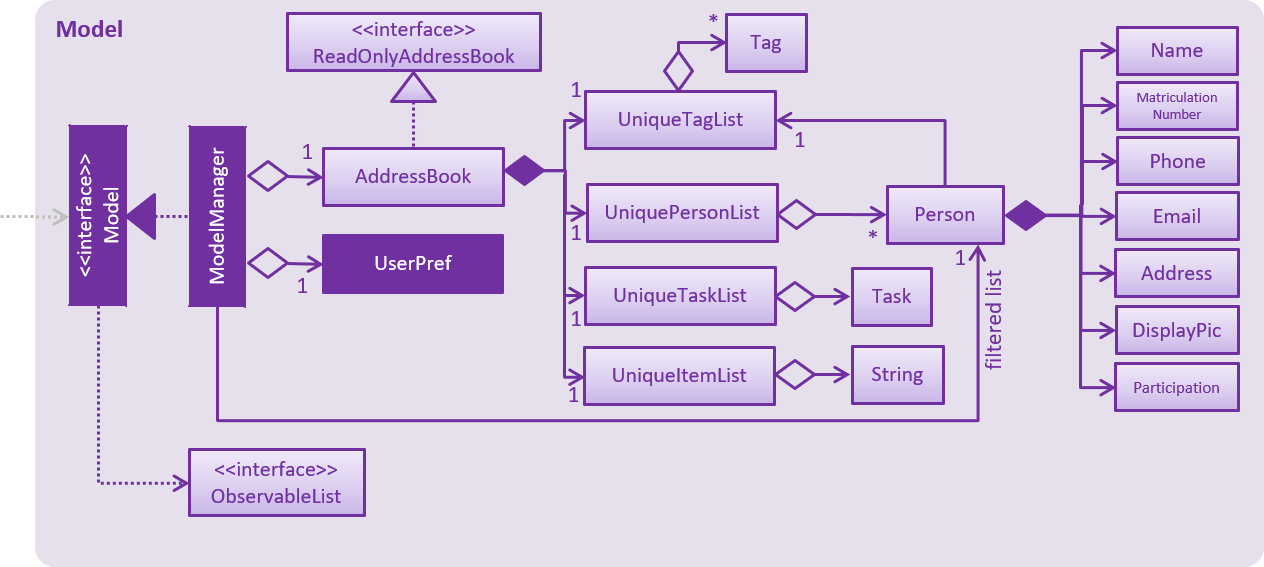
API : Model.java
The Model
:
-
Stores a
UserPref
object that represents the user’s preferences. -
Stores the data used by Your TA.
-
Exposes an unmodifiable
ObservableList<Person>
andObservableList<Task>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
Does not depend on any of the other four major components.
The following are details of the purpose and function of the different classes that make up the entire Model
.
The Person
Class:
-
Stores the information of a specific person (student) in Your TA.
-
Information includes: Name, Matriculation Number, Phone Number, Email, Address, DisplayPic, Participation and different tags to associate with that person.
-
Implements
UniquePersonList
that enforces uniqueness of its elements and disallows nulls. -
Name
: Object that stores the name of thePerson
object.Person’s name should only contain alphanumeric characters, and should not be null. -
Matriculation Number
: Object that stores the matriculation number of thePerson
object.Matriculation number should start with either 'A' or 'U', followed by 7 digits and ending with an alphabet (A-Z). -
Phone Number
: Object that stores the phone number of thePerson
object. -
Email
: Object that stores the email address of thePerson
object.Email address should be of the format local-part@domain. -
DisplayPic
: Object that contains the filepath to the display picture used by thePerson
object. -
Participation
: Object that stores the participation marks of thePerson
object.
The User
Class:
-
Stores the information of a specific user (TA/Lecturer/Professor) in the application.
-
Information includes: Username and Password.
-
Implements
UniqueUserList
that enforces uniqueness of its elements and disallows nulls. -
Username
: Object that stores the username of theUser
Object and contains the regex requirements for a valid username.User’s username should only contain alphanumeric characters, be between 3 and 15 characters long and should not be null. -
Password
: Object that stores the password of theUser
Object and contains the regex requirements for a valid password.User’s password should only contain alphanumeric characters, be between 8 and 30 characters long and should not be null.
The Tag
Class:
-
An immutable object that has to be valid.
-
Checks are implemented to guarantee validity.
For every Person
object, there can be multiple (or zero) tags.
The Task
Class:
-
Stores the information of a specific Task in Your TA.
-
Information includes: Title, Description, Deadline, Priority.
-
Implements
UniqueTaskList
that enforces uniqueness of its elements and disallows nulls. -
Title
&TaskDescription
: Object that stores the title and description of theTask
Object.Tasks title and description should only contain alphanumeric characters, and should not be null. -
Deadline
: Object that stores the deadline of theTask
Object.Deadline should be a valid date that exists and in the format dd-mm-yyyy. Tasks cannot be scheduled in the past. And can only be scheduled at most 6 months in advance. (Based on months: tasks cannot be scheduled on 1st August 2018 if the current date is 31st January 2018). -
Priority
: Object that stores the priority of theTask
Object.Priority value input can only be a value from 1 to 3. 1 being lowest priority and 3 being highest.
4.6. Storage component
The storage component maintains the reading and writing of data used by Your TA. It allows the application to save and read files on the user’s computer.
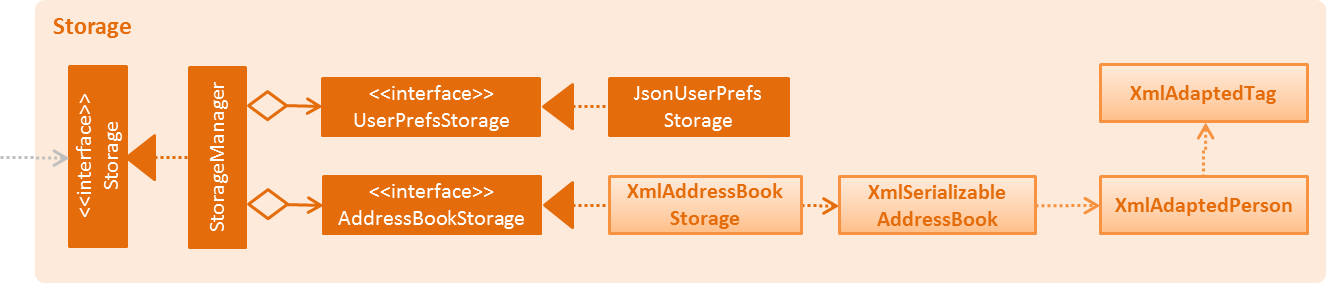
API : Storage.java
The Storage
component:
-
can save
UserPref
objects in json format and read it back. -
can save data used by Your TA in xml format and read it back.
-
can save images used by Your TA.
-
can save the user data in xml format and read it back.
-
can save list of
User
objects for login authentication.
5. Implementation
This section describes some noteworthy details on how certain features are implemented.
5.1. Login feature
5.1.1. Current implementation
The login feature is initialized upon startup of the application, through LoginStorage
and LoginManager
and is facilitated by the LoginUi
.
It supports multiple accounts whereby one user cannot access the application content of another user by creating multiple .xml
storage files.
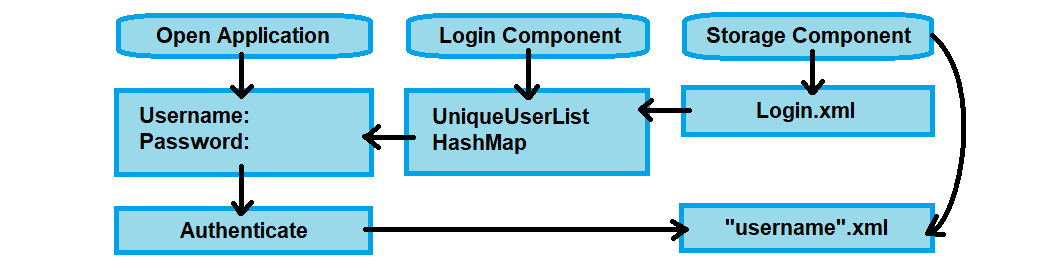
As seen from the Figure 12, upon opening the application, the user is prompted to enter their Username
and Password
. The LoginManager
fetches the data of existing User
objects, before putting them into a Hashmap<Username, User> as shown below.
public synchronized void addUser(String username, String password) throws DuplicateUserException {
if (!userList.getUserList().containsKey(username)) {
Username addUsername = new Username(username);
Password addPassword = new Password(password);
User toAdd = new User(addUsername, addPassword);
userList.add(toAdd);
}
}
Username and Password are case-sensitive.
|
The credentials entered by the user are then checked against the HashMap to authenticate the account. Upon successful login, the user’s file is retrieved from the Storage
component and is loaded up with the application.
@Override
public void authenticate(String username, String password) throws DuplicateUserException {
logger.fine("Authenticating user: " + username);
String filepath = username + ".xml";
if (userList.getUserList().containsKey(username)) {
if (userList.getUserList().get(username).getPassword().getPassword().equals(password)) {
loginUser(filepath);
} else {
throw new DuplicateUserException();
}
} else {
addUser(username, password);
try {
File file = new File("data/login/" + filepath);
file.createNewFile();
} catch (IOException e) {
throw new DuplicateUserException();
}
loginUser(filepath);
}
}
If the user is a new (username does not exist), they should simply enter their desired Username and Password into the respective fields, and the account will be created with the default data of the application.
|
5.1.2. Design considerations
Aspect: Implementing new User
-
Alternative 1 (current choice): Using same login window, create new
User
ifUsername
entered does not exist-
Pros: Use of only 1 window, no need to implement additional UI functionalities.
-
Cons: Not the most user-friendly or the most conventional way a login works.
-
-
Alternative 2: Create a registration button, which brings the user to a registration UI for the creation of
User
object-
Pros: More user-friendly, able to implement a username field, along with a password field and, especially a field for password confirmation.
-
Cons: Need to further add onto UI.
-
Aspect: Managing existing users
-
Alternative 1 (current choice):
User
is immutable-
Pros: Easier to keep track of
User
. -
Cons: Highly inflexible, not user-friendly.
-
-
Alternative 2:
User
to be mutable, can be edited or deleted-
Pros: Users can change their usernames/passwords if they feel their credentials may be insecure.
-
Cons: Need for rigorous salting/hashing if repeated usernames/passwords are allowed.
-
Aspect: User
identification
-
Alternative 1 (current choice):
Username
is case-sensitive-
Pros: "Johndoe" and "johndoe" are different usernames and different accounts with the same name can be created.
-
Cons: If a user makes a typo in the
Username
field, another account is created instead of correctly logging on to their account.
-
-
Alternative 2:
Username
to be made case-insensitive-
Pros: Users will not have to worry about whether they signed up with a different
Username
. -
Cons: Less usernames are available.
-
Aspect: Username
and Password
representation
-
Alternative 1 (current choice):
Username
andPassword
only allow alphanumeric characters-
Pros: Easy authentication, no need to worry about corner cases.
-
Cons: Less room for different usernames and passwords, not as secure.
-
-
Alternative 2: Include special characters in
Username
andPassword
Regex-
Pros: More secure, less prone to security issues.
-
Cons: Need for more rigorous testing to ensure no corner cases are left out.
-
Aspect: Salting passwords/encryption of files
-
Alternative 1 (current choice): No encryption/salting implemented
-
Pros: Ease of editing information by administrator.
-
Cons: Security issues, easy to find data path and retrieve files.
-
-
Alternative 2: Encrypt files and salt passwords before encryption
-
Pros: Increases security of software, less prone to be used for malicious purposes.
-
Cons: Large amount of coding and implementation required, prerequisites also include knowledge of security issues and safeguards.
-
5.2. Undo/Redo feature
5.2.1. Current implementation
The undo/redo mechanism is facilitated by an UndoRedoStack
, which resides inside LogicManager
. It supports undoing and redoing of commands that modifies the state of the address book (e.g. add
, edit
). Such commands will inherit from UndoableCommand
.
UndoRedoStack
only deals with UndoableCommands
. Commands that cannot be undone will inherit from Command
instead. The following diagram shows the inheritance diagram for commands:
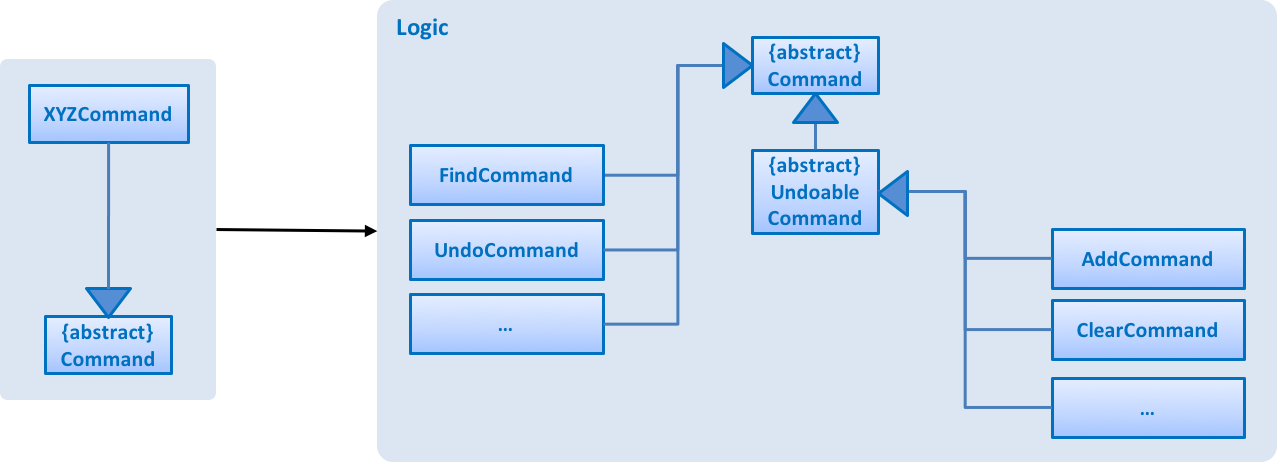
As you can see from the diagram, UndoableCommand
adds an extra layer between the abstract Command
class and concrete commands that can be undone, such as the DeleteCommand
. Note that extra tasks need to be done when executing a command in an undoable way, such as saving the state of the address book before execution. UndoableCommand
contains the high-level algorithm for those extra tasks while the child classes implements the details of how to execute the specific command. Note that this technique of putting the high-level algorithm in the parent class and lower-level steps of the algorithm in child classes is also known as the template pattern.
Commands that are not undoable are implemented this way:
public class ListCommand extends Command {
@Override
public CommandResult execute() {
// ... list logic ...
}
}
With the extra layer, the commands that are undoable are implemented this way:
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class DeleteCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... delete logic ...
}
}
Suppose that the user has just launched the application. The UndoRedoStack
will be empty at the beginning.
The user executes a new UndoableCommand
, delete 5
, to delete the 5th person in the address book. The current state of the address book is saved before the delete 5
command executes. The delete 5
command will then be pushed onto the undoStack
(the current state is saved together with the command).

As the user continues to use the program, more commands are added into the undoStack
. For example, the user may execute add n/David …
to add a new person.
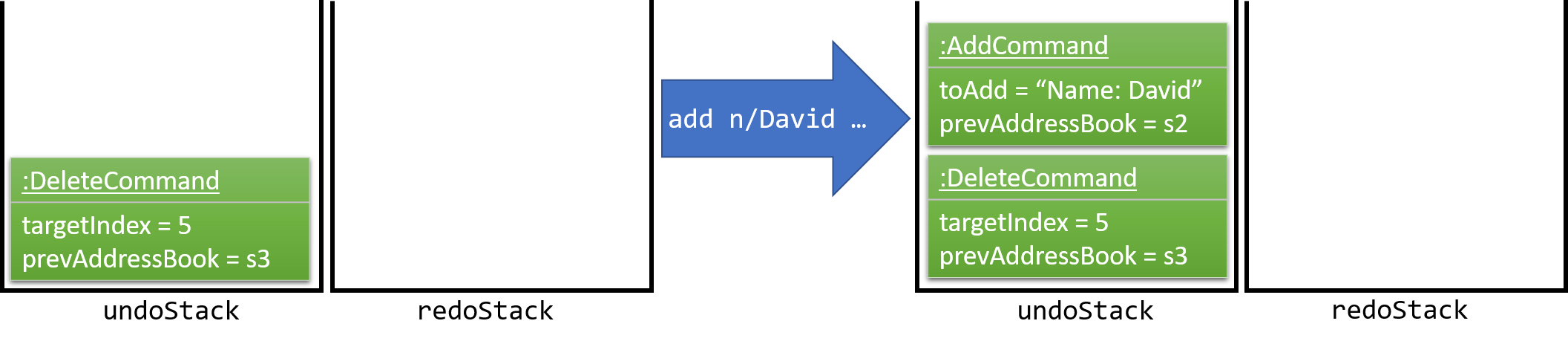
If a command fails its execution, it will not be pushed to the UndoRedoStack at all.
|
The user now decides that adding the person was a mistake, and decides to undo that action using undo
.
We will pop the most recent command out of the undoStack
and push it back to the redoStack
.
It would then proceed to restore the address book to the state before the add
command executed.
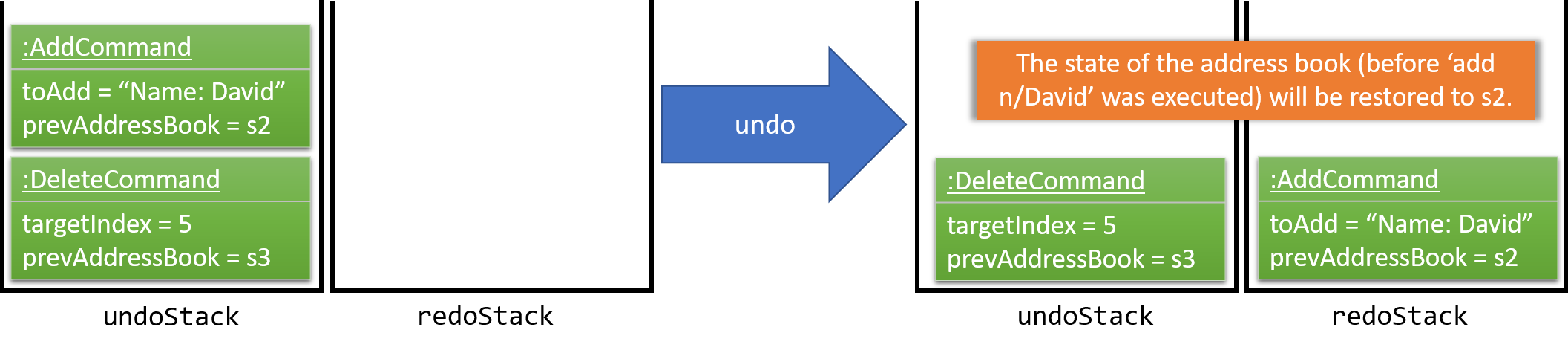
If the undoStack is empty, then there are no other commands left to be undone, and an Exception will be thrown when popping the undoStack .
|
The following sequence diagram shows how the undo operation works:
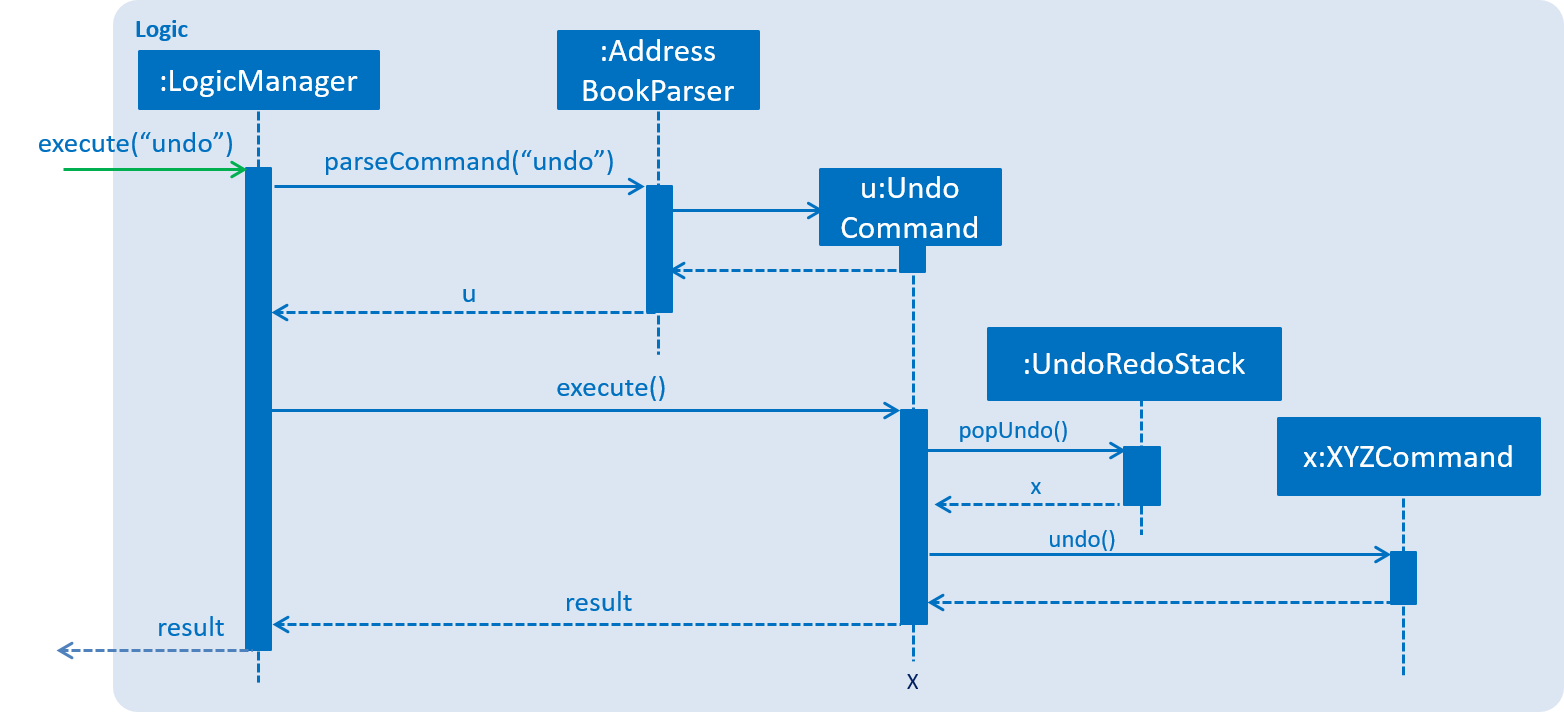
The redo does the exact opposite (pops from redoStack
, push to undoStack
, and restores the address book to the state after the command is executed).
If the redoStack is empty, then there are no other commands left to be redone, and an Exception will be thrown when popping the redoStack .
|
The user now decides to execute a new command, clear
. As before, clear
will be pushed into the undoStack
. This time the redoStack
is no longer empty. It will be purged as it no longer make sense to redo the add n/David
command (this is the behavior that most modern desktop applications follow).

Commands that are not undoable are not added into the undoStack
. For example, list
, which inherits from Command
rather than UndoableCommand
, will not be added after execution:

The following activity diagram summarize what happens inside the UndoRedoStack
when a user executes a new command:
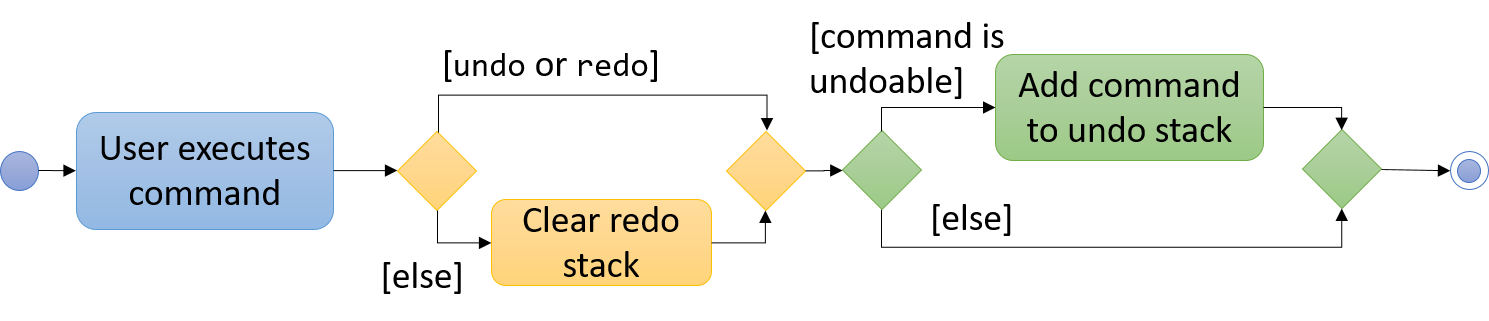
5.2.2. Design considerations
Aspect: Implementation of UndoableCommand
-
Alternative 1 (current choice): Add a new abstract method
executeUndoableCommand()
-
Pros: We will not lose any undone/redone functionality as it is now part of the default behaviour. Classes that deal with
Command
do not have to know thatexecuteUndoableCommand()
exist. -
Cons: Hard for new developers to understand the template pattern.
-
-
Alternative 2: Just override
execute()
-
Pros: Does not involve the template pattern, easier for new developers to understand.
-
Cons: Classes that inherit from
UndoableCommand
must remember to callsuper.execute()
, or lose the ability to undo/redo.
-
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire address book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the person being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
Aspect: Type of commands that can be undone/redone
-
Alternative 1 (current choice): Only include commands that modifies the address book (
add
,clear
,edit
).-
Pros: We only revert changes that are hard to change back (the view can easily be re-modified as no data are * lost).
-
Cons: User might think that undo also applies when the list is modified (undoing filtering for example), * only to realize that it does not do that, after executing
undo
.
-
-
Alternative 2: Include all commands.
-
Pros: Might be more intuitive for the user.
-
Cons: User have no way of skipping such commands if he or she just want to reset the state of the address * book and not the view. Additional Info: See our discussion here.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use separate stack for undo and redo
-
Pros: Easy to understand for new Computer Science student undergraduates to understand, who are likely to be * the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update * both
HistoryManager
andUndoRedoStack
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate stack, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two * different things.
-
5.3. Matriculation number
This feature allows the user to keep track of the matriculation number of a Person
. The matriculation number in this case
has to start with an "A" or "U" followed by 7 digits and end with a capital letter. A new class, Matriculation Number
, is created and is associated to the Person
class.
This is integrated into the AddCommand
and EditCommand
commands to update the participation marks of a Person
.
5.3.1. Current implementation
The user will input an AddCommand or EditCommand, with the additional parameter m/MATRICULATION_NUMBER
, to the application to update the marks.
5.3.2. Design considerations
Aspect: Should 2 people be allowed to have to same matriculation number
-
Alternative 1 (current choice): No, no 2 people can have the same matriculation number, DuplicateUserException will be thrown when trying to add a person with a matriculation number already inside Your TA.
-
Pros: It makes sense as no 2 students would have the same matriculation number, it alerts the user when they’ve most likely typed in the wrong matriculation number.
-
Cons: The user will not be able to add 2 different instances of the same student.
-
-
Alternative 2: Duplicate matriculation numbers are allowed
-
Pros: User have more freedom to add anyone.
-
Cons: Duplicate matriculation numbers will cause messiness when dealing with many students.
-
5.3.3. Future enhancements (Coming in v2.0)
-
Link matriculation number to the students IVLE account info.
5.4. Participation
This feature allows the user to keep track of participation marks of a Person
. The participation marks in this case is limited to an integer value of 0 to 100 inclusive. A new class, Participation
, is created and is associated to the Person
class.
This utilizes the MarkCommand
command to update the participation marks of a Person
.
5.4.1. Current implementation
The user will input a command, markPart INDEX marks/DIGITS
, to the application to update the marks.
The following figure 13 and paragraph below shows the sequence of how the MarkCommand
command functions:
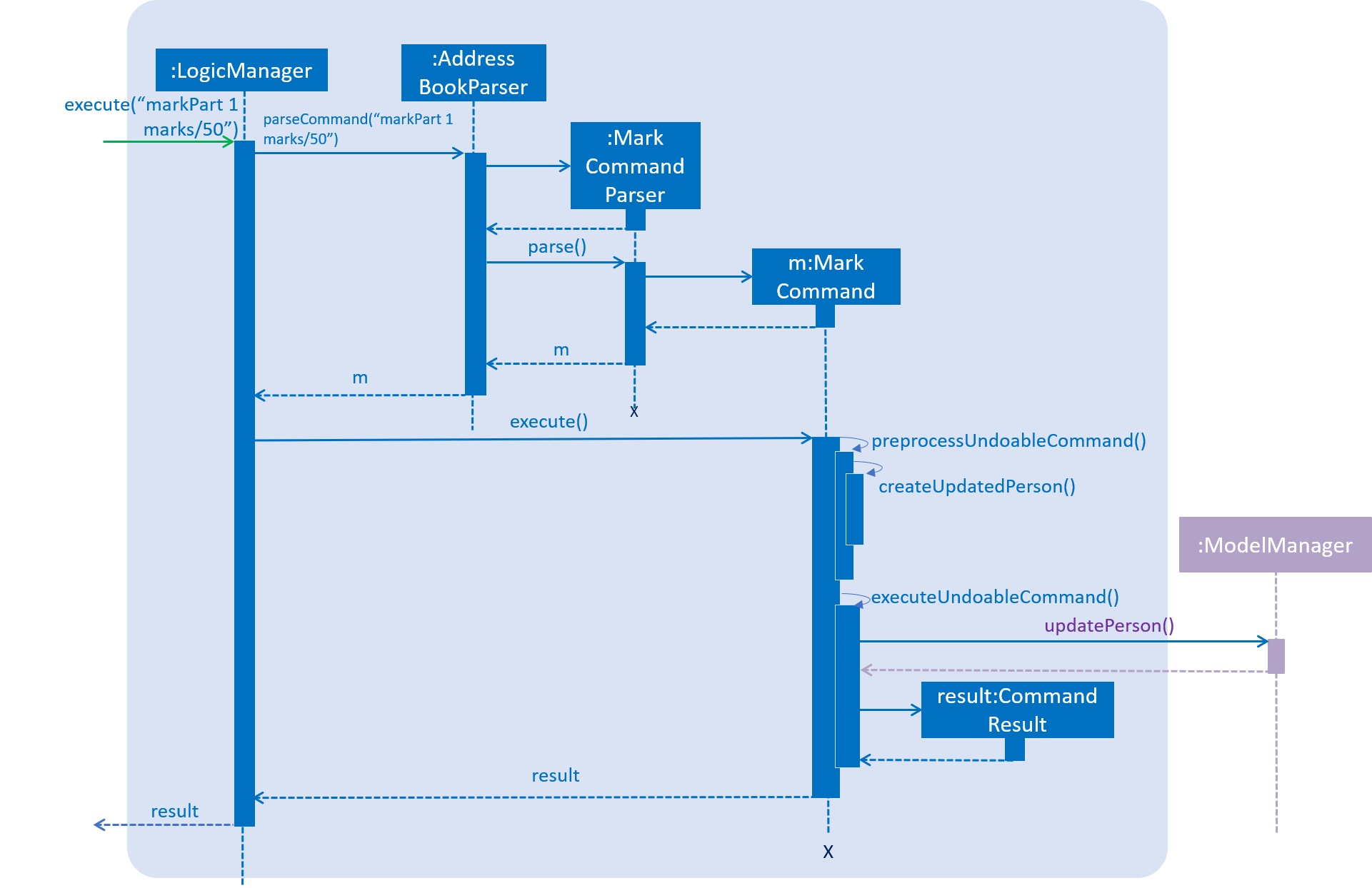
-
The user will enter the command
markPart INDEX marks/DIGITS
to the application. -
The application will then pass the arguments to
AddressBookParser
which in turns passes it toMarkCommandParser
to parse the argument. -
The
MarkCommandParser
would then create aMarkCommand
with the data from the arguments. -
The
LogicManager
will then execute thepreprocessUndoableCommand()
inMarkCommand
. -
The
preprocessUndoableCommand()
will then execute thecreateUpdatedPerson()
to create a newPerson
object with a newParticipation
object containing the new total marks. -
Finally, this new
Person
object created in step 3 will replace the originalPerson
object with the oldParticipation
object stored in theModel
through theupdatePerson()
method.
5.4.2. Design considerations
Aspect: How to update the marks
-
Alternative 1 (current choice): Create an entire new
Person
object-
Pros: It is similar to the rest of the
Logic
commands. -
Cons: This uses more memory when executing.
-
-
Alternative 2: Make the
value
in theParticipation
class editable-
Pros: It uses less memory, and only the value has to be updated.
-
Cons: The implementation will require the writing of more methods.
-
Aspect: Setting a limit to the number of marks
-
Alternative 1 (current choice): Set the limit of participation marks to 100
-
Pros: Maintain a fixed value, prevents unforeseen issues such as integer overflows.
-
Cons: The user is restricted to only 100 marks total, and has to be more careful in the amount of marks they wish to add.
-
-
Alternative 2: Do not set a limit for the amount of participation marks
-
Pros: It is more flexible, and allows more variation in the marks the user wishes to add
-
Cons: This requires more validation checks to prevent issues like an integer overflow.
-
5.4.3. Future enhancements (Coming in v2.0)
-
Support for setting a threshold and easily seeing how many students made the cut over the threshold.
5.5. Display picture
Users are able to add a display picture for any person within the application. The user can utilise 3 different commands (add
, edit
and updateDP
) to create and specify a display image for the person.
It fully supports the undo
and redo
commands. It adds a drop shadow around the frame to indicate the level of participation (see above section 5.4 Participation) of the person.
This feature allows the user to enter a path to their selected image file when entering any of the above 3 commands and copies the image into the data/displayPic
folder.
It utilises the DisplayPicStorage
class to handle all image storage related operations.
It also makes use of the Participation
feature to display a colored shadow around the display picture.
This image will be shown in the application next to the details of the person as seen in figure 14 below.
5.5.1. Current implementation
An additional class, DisplayPic
, is added to the Person
class. This class contains the filepath to the stored image file.
It uses validation checks to ensure that the image meets the following requirements:
-
It is a file that exists and has a file extension.
-
It is a valid image file that can be opened as an image.
If it passes the checks, then a new DisplayPic
object will be created with the filepath to the image stored as its value.
It will then proceed to duplicate the image. After duplicating the image, the value will be further updated with the duplicate image’s filepath.
Adding a display picture when creating a new person
The add
command supports a new field dp/
, where the user will provide the filepath of the image and the newly created Person
will have that specified image as the display picture.
For persons that were not specified a dp/
during the add
command, the DisplayPic
object associated to them would contain the value of the default display picture.
Editing a display picture
The display picture can also be changed by using the edit
or updateDP
commands.
The implementation of the UpdateDisplayCommand (updateDP
) command closely follows the edit
command, hence we will only showcase the implementation of the UpdateDP
command.
The following figure 15 is the sequence diagram of the updateDP
command to show how it functions:
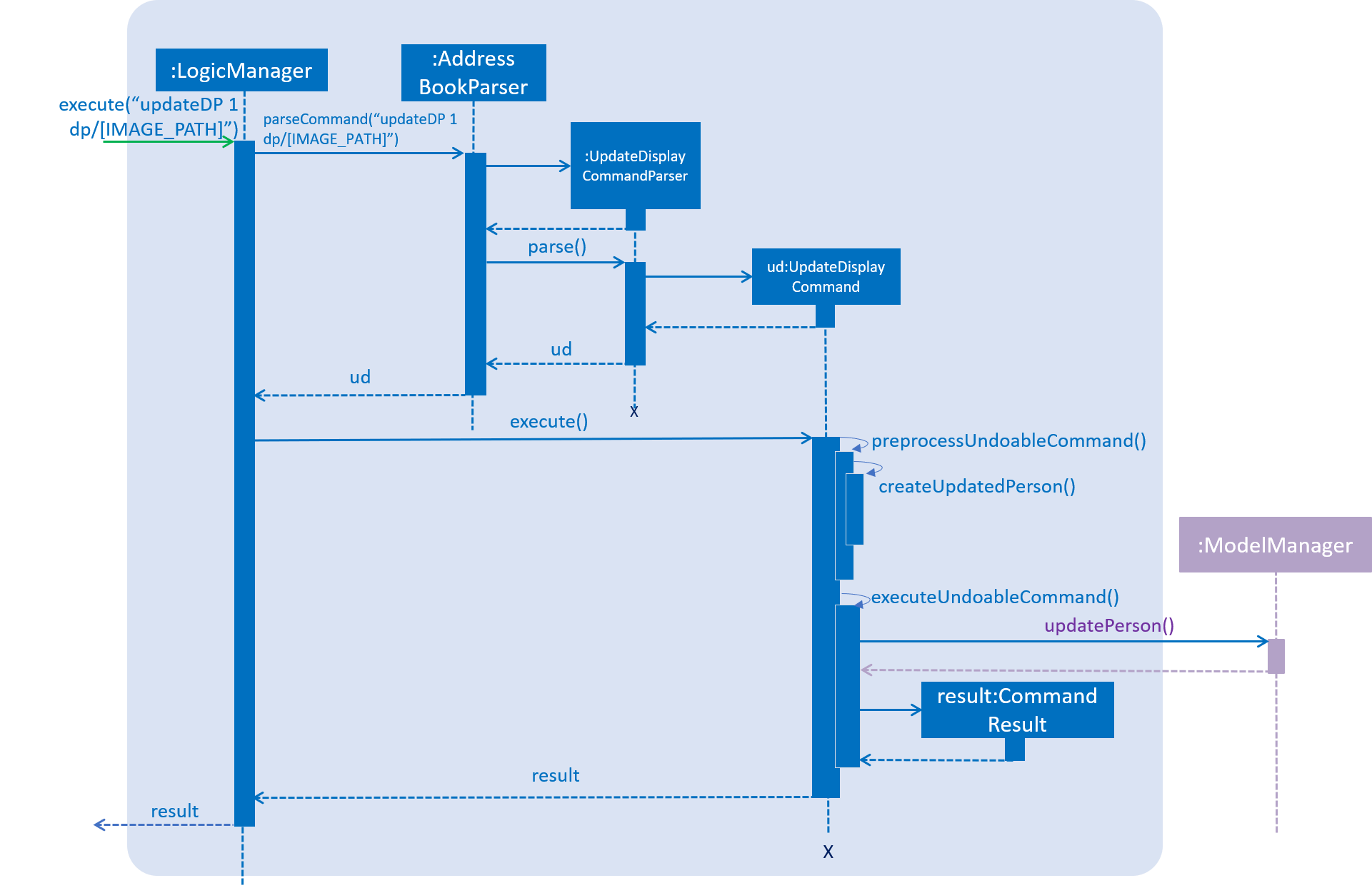
-
The user input will be passed in and parsed by the
AddressBookParser
andUpdateDisplayCommandParser
. -
The
UpdateDisplayCommandParser
then creates a newUpdateDisplayCommand
where theLogicManager
executes thepreprocessUndoableCommand()
. -
The
preprocessUndoableCommand()
will execute thecreateUpdatedPerson()
to create a newPerson
object with the updatedDisplayPicture
object which contains the new filepath. -
Finally, this new
Person
object created in step 3 will replace the originalPerson
object with the oldDisplayPic
object stored in theModel
through theupdatePerson()
method.
Deleting a display picture
To fully support the undo
and redo
commands, image files cannot be immediately deleted when it is not in use by the UI
.
To work around this, a new class UniqueItemList
was added to the model of the AddressBook
as seen below in Figure 16.
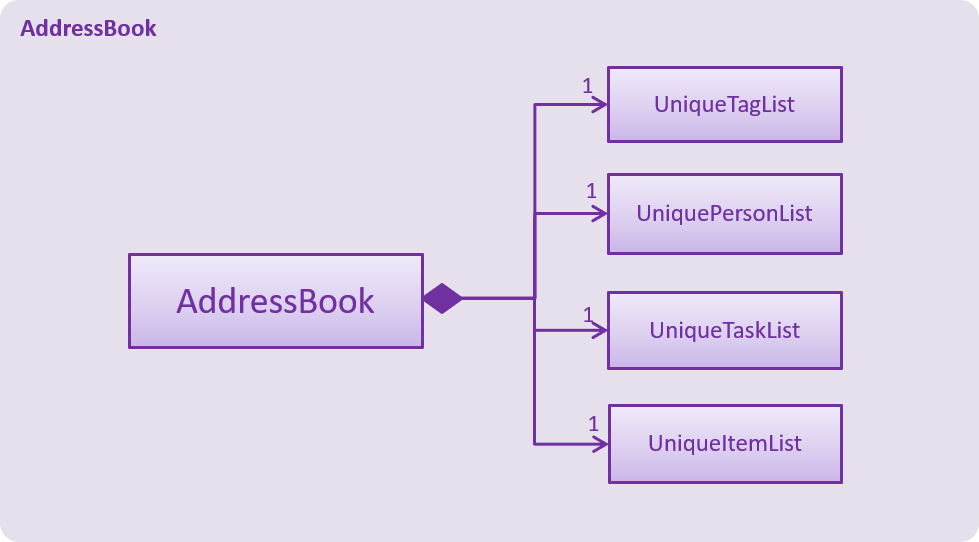
The UniqueItemList
consists of an ArrayList
of unique String
objects. These String
objects represent the filepaths of all images that have been added or are used by the application during its runtime.
Upon every launch of the application, during the initialization of the LogicManager
class, it will run through the UniqueItemList
and delete
any unused image files from the data
folder. It does this by looping through the UniquePersonList
as well and checks if the image file is used. If it is not used, it will be deleted.
The following code fragment shows the deletion process:
public static void clearImageFiles(List<String> itemsToDelete, ObservableList<Person> persons) {
for (String item : itemsToDelete) {
boolean notUsed = true;
for (Person p : persons) {
if (p.getDisplayPic().toString().equals(item) || item.equals(DisplayPic.DEFAULT_DISPLAY_PIC)) {
notUsed = false;
break;
}
}
if (notUsed) {
//deletion of file occurs here
}
}
}
The list is then cleared for the next usage of the application.
Storing the image file for the display picture
The application will take in an argument for the 3 commands mentioned above through dp/ [PATH TO IMAGE]
. The [PATH TO IMAGE]
can be the absolute or relative path to the image file.
An example of a [PATH TO IMAGE] would be C:\Users\Desktop\Image.jpg for Windows.
|
If this [PATH TO IMAGE]
leads to a non-existent file or a non-image file, it will utilise the default profile picture
which is stored in src/resources/images/displayPic
as default.png
.
If a valid path to an image is provided, the image will be processed and copied over to the data
folder where the addressbook.xml
is stored as well.
The filename of the image copied over will be a SHA-256 hash. This hash is calculated over the Person
object’s details (i.e Name, Phone and Email) to ensure a unique filename.
The following activity diagram in Figure 17 shows how the files are saved:
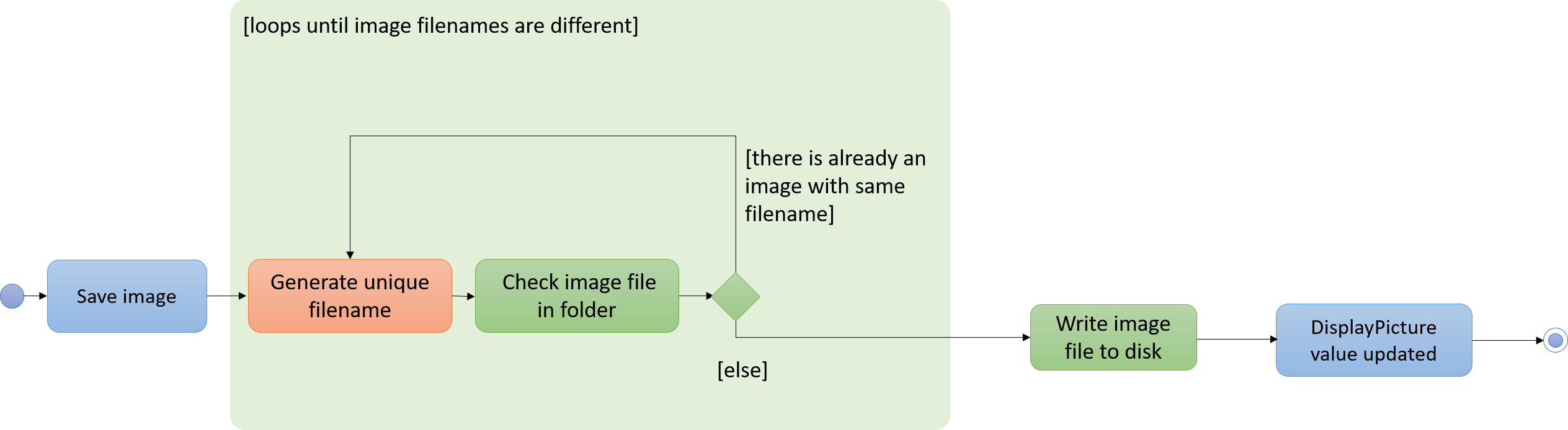
Due to the possibility that display pictures for a Person
can be updated, this could lead to clashing of the same file names. In order to prevent overwriting of files, the new image filename
will be the SHA-256 hash of the previous image filename.
The code utilised to duplicate the image file copies the file byte for byte, ensuring that they are binary equivalent. The code used is implemented as follows:
public static void copyFile(String origFile, File outputFile) throws IOException {
//initialize buffer
//open bis/bos as the buffered input and output streams respectively
int fileBytes = bis.read(buffer);
while (fileBytes != -1) {
bos.write(buffer, 0, fileBytes);
fileBytes = bis.read(buffer);
}
//close IO streams
}
Finally, the DisplayPic
object will then be updated to store the relative filepath to this new duplicated image.
Fetching and displaying the image file
The following activity diagram shows the flow of how an image file is retrieved to be displayed.
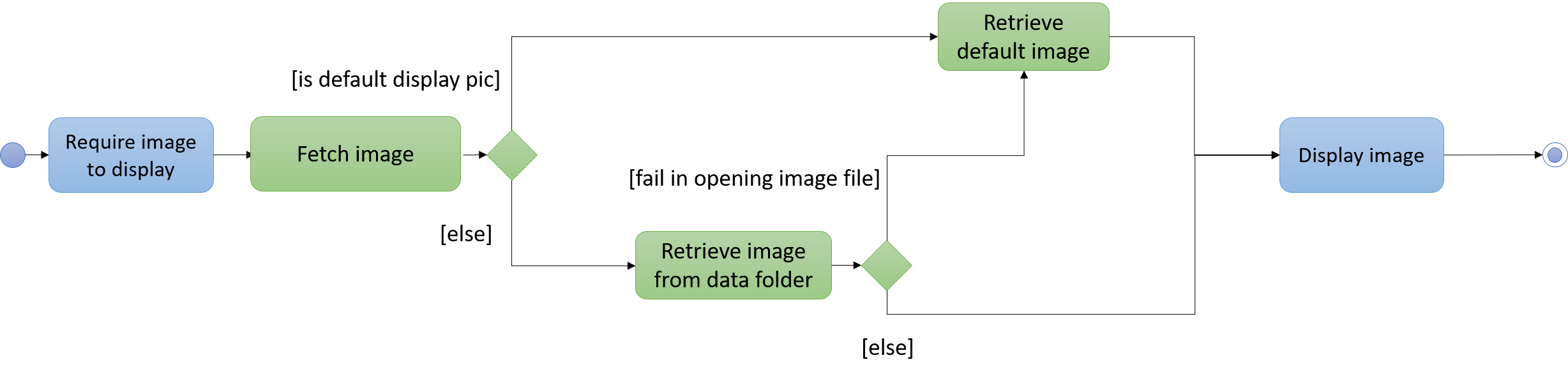
The image file will then be utilized by JavaFX and displayed on the UI. The drop shadow of the image is determined by the Participation
of the Person
.
5.5.2. Design considerations
Aspect: Filepath to the image
-
Alternative 1 (current choice): Copy the image file over to a designated location.
-
Pros: The user does not need to maintain the image file, as the application does so itself.
-
Cons: Duplication of the image file will take up more space on the user’s storage.
-
-
Alternative 2: Use the location of the file the user enters into the application.
-
Pros: This would mean that there would always be only one copy of the image, hence less space used.
-
Cons: The user needs to ensure the original image file is not moved or deleted.
-
Aspect: Filename of the image
-
Alternative 1 (current choice): Use a hashing algorithm to name the file.
-
Pros: SHA-256 provides collision resistance which means the filename would be unique majority of the time and it is easy to implement.
-
Cons: It is difficult to manually check which
Person
the image file belongs to.
-
-
Alternative 2: Use the name of the
Person
to name the file.-
Pros: Image files can be easily identified separately and easy to implement.
-
Cons: This could lead to potential image files overwriting each other without additional checks.
-
Aspect: Deletion of the image
-
Alternative 1 (current choice): Store the filepath in the
AddressBook
and delete it afterwards.-
Pros: Fully supports
undo
andredo
even if the user moves or deletes the original image file. -
Cons: It is difficult to manually check which
Person
the image file belongs to.
-
-
Alternative 2: Delete the image file immediately when the associated
Person
is removed.-
Pros: This allows a simple implementation of immediately deleting the image file.
-
Cons: The image file could be lost forever if the user moves or deletes the original image file, thus causing
undo
andredo
to malfunction.
-
5.5.3. Future enhancements (Coming in v2.0)
-
Support for online URLs to download images.
5.6. Task list
Users are able to add, edit and remove tasks (with command addTask
, editTask
and deleteTask
) in order to schedule their tasks within the application.
Tasks scheduled in this application will be stored in addressbook.xml
and displayed in the Todo List
tab and Calender
view respectively.
Todo List
displays tasks with all fields (title, description, deadline and priority) in a list view whereas Calender
displays tasks with only task titles in their respective date cells determined by their deadline.
Tasks displayed in a date cell are color coded according to their priorities — Green being lowest, yellow being medium and red being highest . Tasks are displayed in Todo List
and Calender
as shown in figure 19.
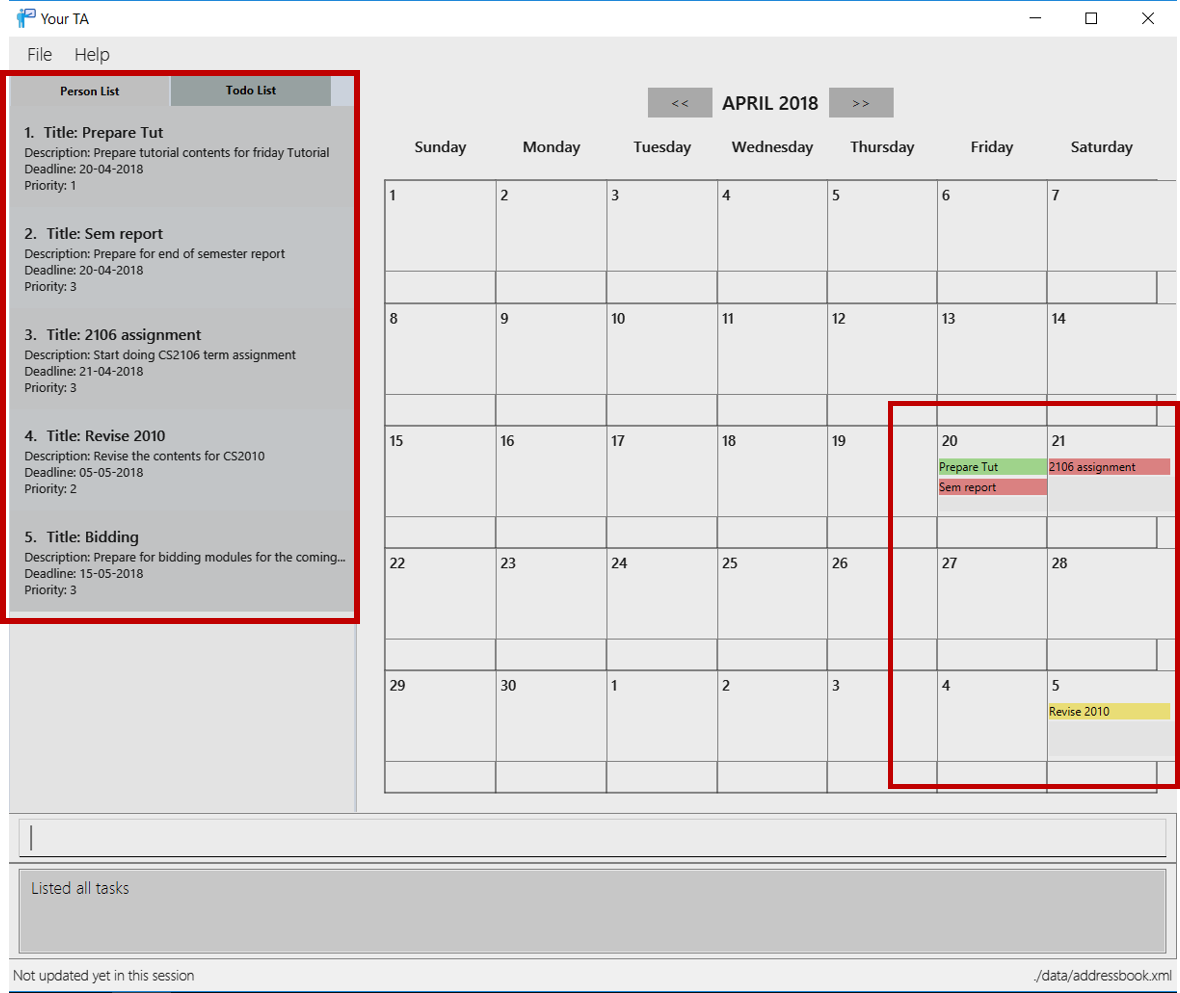
5.6.1. Current implementation
An additional class, UniqueTaskList
is added to the AddressBook
class. This class contains an ObservableList
of tasks and a 2-dimensional ObservableList
of tasks.
The previous is the actual copy of tasks stored in addressbook.xml
while the latter is a copy of reorganized tasks for displaying in Calendar
.
Adding a task
The addTask
command requires fields of title/
, desc/
, by/
and priority/
to be present.
Any missing field will cause a built-in exception thrown when creating the Task
object as the addTaskCommandParser
validates the presence of all required fields.
The exception prevents the invalid task from being created and added into UniqueTaskList
.
Multiple of same field is allowed but all are discarded except the last entry of that field by ArgumentTokenizer
called in AddTaskCommandParser
.
After successful creating the Task
object, ModelManager
will help add the object into Addressbook
.
Deleting a task
The deleteTask
command requires a positive task index which specifies the task to be deleted. The DeleteTaskCommandParse
validates this index with accordance to the current displayed Todo List
size.
If the index is valid, the DeleteTaskCommand
will delete the corresponding Task
object from UniqueTaskList
.
Editing a task
The editTask
command requires a positive task index and desired edit fields indicated by prefix title/
, desc/
, by/
and priority/
. The EditTaskCommandParser
validates that there is at least one field specified in the command.
EditTaskCommand
then creates a new Task
object with modified fields and other unmodified fields identical.
The AddressBook
will be called by ModelManager
to delete the original Task
object from the UniqueTaskList
and add the newly created task to the UniqueTaskList
.
Add task, delete task and edit task commands support |
Add task, delete task and edit task commands have similar sequence behavior. Below is an example of the overview how components interact during deletion of a task.
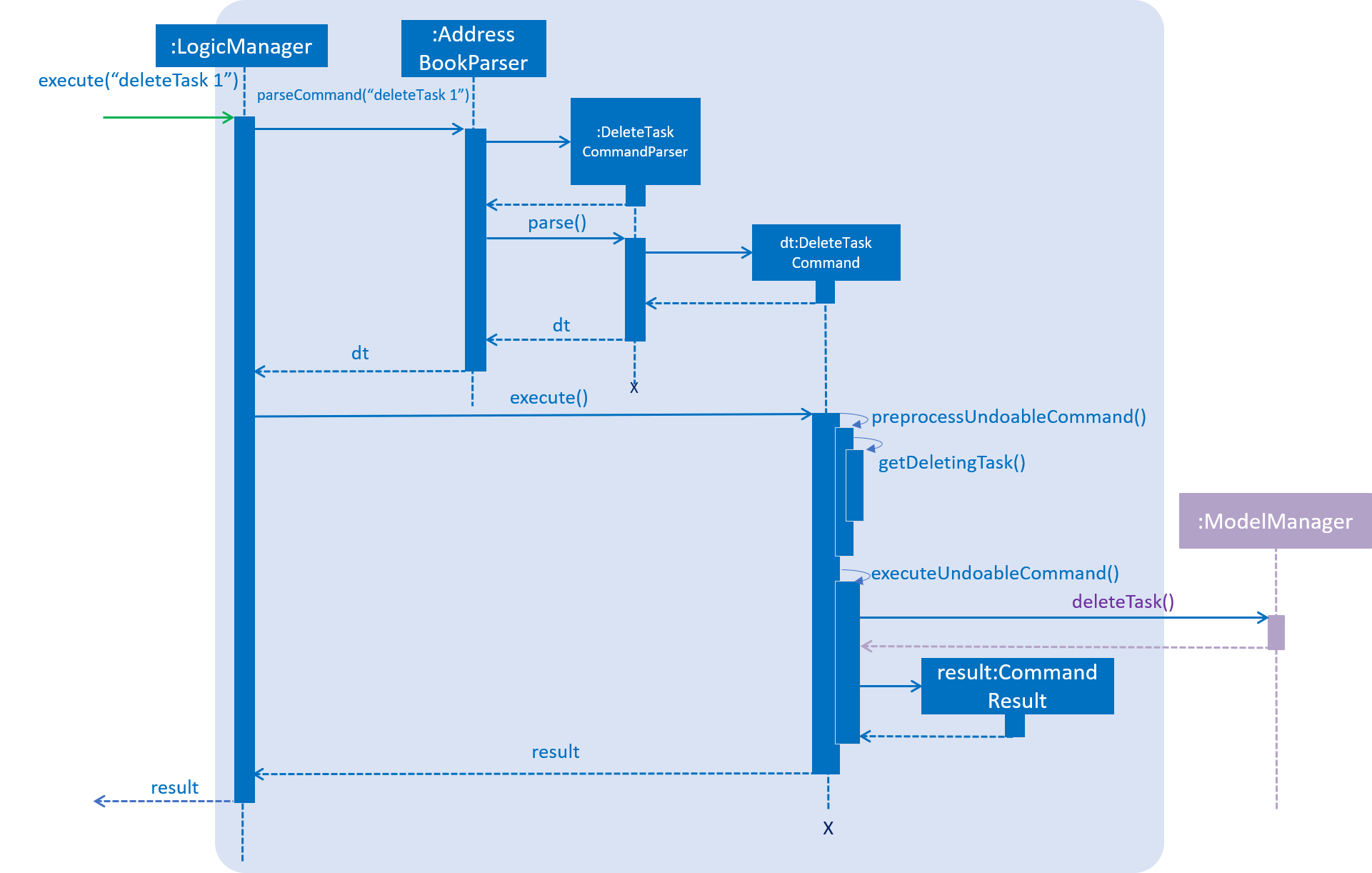
Listing tasks
The execution of listTask
command parses a predicate argument PREDICATE_SHOW_ALL_TASK to ModelManager
which updates the task list based on this predicate.
This will extract all tasks saved in the data file addressbook.xml
.
Listing current month tasks
The execution of listCurrentTask
command parses the predicate argument PREDICATE_SHOW_ALL_CURRENT_TASK to Model
. ModelManager
then updates the task list based on this predicate.
This is similar to Listing tasks but it will only extract tasks with deadlines due in the current month.
Sorting tasks
Task
class implements Java Comparable<>
class which enables sorting without writing the actual sorting algorithm. Thus,the execution of sortTask
command relies on it.
Model
then calls AddressBook
which sorts the tasks in UniqueTaskList
.
The sorting is done by comparing the year, month and date sequentially. This is specified in the compareTo() method in Task
.
listing and sorting tasks do not support |
5.6.2. Design consideration
Aspect: How EditTaskCommand
executes
-
Alternative 1(current choice): Adds a new Task and deletes the original task
-
Pros: Easy to implement, no need to worry about the change of deadline shown in
Calendar
-
Cons: Unable to maintain the original task list order.
-
-
Alternative 2: Edits the field of original task
-
Pros: Maintains the task list order
-
Cons: Difficult to implement as a new mechanism to sync the
Calendar
cells is needed.
-
5.7. Tasks on calendar view
-
This feature allows the user to see the calendar on the UI and see the scheduled
Task
deadlines on the calendar.
5.7.1. Current implementation
The user will enter a command addTask title/TITLE desc/DESCRIPTION by/DEADLINE priority/PRIORITY
to the application. The application will then rely on AddressBookparser
and
parse the argument. The argument will be passed into the AddTaskCommand to be executed. From there, it will create a new Task
object that will be
added to the calendarList
of the UniqueTaskList
which in turn will be displayed on the date of the deadline, on the calendar.
5.7.2. Design considerations
Aspect: How to link specific tasks to the correct calendar nodes
-
Alternative 1 (current choice): Use a 7 by 32, 2D
Array
to store the tasks to be added into the calendar.-
Pros: Separate from the Task list (less prone to bugs). Faster run time (does not need to iterate through all tasks).
-
Cons: Higher memory usage constraint, can only schedule tasks up to 6 months in advance to prevent high memory usage that will affect the speed and performance of the app.
-
-
Alternative 2: Use the task list itself to be displayed in the calendar.
-
Pros: Easier to program and less memory usage (only 1 task list is used without another 2D array for the calendar), tasks can be scheduled as far ahead as desired.
-
Cons: Needs to iterate through the entire task list for every calendar node when loading the calendar view (slows performance when dealing with tasks).
-
Aspect: Viewing the calendar only by month
-
Alternative 1 (current choice): Use a monthly only calendar view.
-
Pros: Easy view of the current tasks that are due on that month.
-
Cons: Not much choice for the user as only the monthly view is provided.
-
-
Alternative 2: Give the user a choice to switch between weekly, monthly or yearly, e.g. calendarfx.
-
Pros: User has more choice and freedom to control their UI.
-
Cons: Difficult to implement, also if 3rd party programs such at calendarfx is used, certain features in those programs are redundant (e.g. map) and would only slow the app down.
-
5.7.3. Future enhancements (Coming in v2.0)
-
Link specific key-press or select actions to the todo list such that a click on either side will display the selected task on the other.
5.8. Logging
We utilize java.util.logging
package for logging. The LogsCenter
class manages the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file. (See Section 5.9, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level. -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
5.9. Configuration
Certain properties of the application can be controlled (e.g app name, logging level) through the configuration file (default: config.json
).
5.10. Data encryption (coming in v2.0)
{Explain here how the data encryption feature will be implemented}
6. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
6.1. Editing documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
6.2. Publishing documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
6.3. Converting documentation to PDF format
Use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
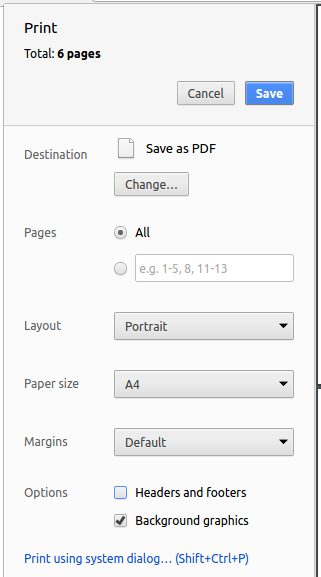
7. Testing
7.1. Running tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
To run the tests, You need to open a console or terminal and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
Detailed information on how to run tests using Gradle is specified in UsingGradle.adoc. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
7.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit Tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit Tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration Tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of Unit and Integration Tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
7.3. Troubleshooting testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
UserGuide.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
8. Dev Ops
8.1. Build automation
See UsingGradle.adoc to learn how to use Gradle for Build Automation.
8.2. Continuous integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
8.3. Coverage reporting
We use Coveralls to track the code coverage of our projects.
See UsingCoveralls.adoc for more details.
8.4. Documentation previews
When there are changes to asciidoc files in a pull request, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged.
See UsingNetlify.adoc for more details.
8.5. Making a release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
8.6. Managing dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Product Scope and Features
A.1. Product scope
Target user profile: Tech-Savvy University Teachers/Tutors
-
have the need to manage a significant number of contacts
-
prefer desktop apps over other types
-
can type fast
-
prefer typing over mouse input
-
are reasonably comfortable using CLI apps
Value proposition: manage contacts faster than a typical mouse/GUI driven app, includes to-do list features (with prioritization, etc.) and scheduling (with calendar)
A.2. Feature contribution
Wu Di
-
Major: Implement the todo list
-
Support adding, editing and deleting of tasks
-
Works with undo/redo functions
-
This enables the tutor to view all tasks in one glance so that he/she is able to stay organized and productive.
-
Minor: Import feature to migrate data from an external file
-
Imports data anywhere in any OS
-
This helps the tutor work on different devices with the unique data set.
-
Minor: add the alias feature to some of the commands
-
Support majority of commands
-
This helps the tutor remember the commands intuitively and type them more efficiently.
Daniel
-
Major: Calendar and Scheduler for user
-
Supports adding of new tasks that will be slotted into the calendar(if it has a deadline)
-
Also assigns a priority value to each task based on parameters keyed in when task is added
-
This helps the tutor to keep track of what needs to be done and which task to focus on.
-
Minor: Person has new parameter - Matriculation number
-
Person now stores matriculation number of the person
-
Add command supports adding person with matriculation number(compulsory parameter)
-
Find command supports search by matriculation number
-
This helps the tutor to easily search for a certain student by their unique matriculation number instead of just their names (e.g. easier than searching for a common name such as Daniel).
-
Minor: Toggle between dark and light theme
-
The app can now be toggled between 2 different themes.
-
This allows the tutor to customise their GUI so that they do not have to be restricted to 1 set layout throughout the entire time they use the app.
Pearlissa
-
Major: Login feature
-
Implements new User package
-
This allows multiple tutors to store their data in separate accounts, which can only be accessed by them.
-
Minor: Sorting of contacts based on selected parameters
-
Any number of parameters (at least 1), and in order of priority
-
This allows tutors to be better able to go through their lists of students.
Ellery
-
Major: Display picture for all students entered
-
Support adding, editing and deleting of picture
-
Also shows a quick glance on student’s participation (related to minor)
-
Also works with the redo/undo function
-
This helps the tutor keep track of his students, and be able to easily remember them better.
-
Minor: Participation marks to keep track of student participation
-
Add and keep track of participation marks for the student
-
This helps the tutor to keep track of the student’s participation.
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
new user |
get error messages/prompts |
better adapt to commands to use them properly |
|
user |
add a new person |
better manage all my contacts at one go |
|
user |
delete a person |
remove entries that I no longer need |
|
user |
find a person by name |
locate details of persons without having to go through the entire list |
|
user |
find a person by matriculation number/email |
identify people easily |
|
user |
sort contacts based on name/address/email/tags |
work with specific groups of people |
|
user |
assign a to-do list to each person in address book |
know what I need to do for them |
|
user |
add individual items to the to-do lists |
update additional tasks |
|
user |
remove entire to-do lists or items in it |
remove completed tasks |
|
user |
add events to the schedule |
better manage work/students |
|
user |
add a deadline to tasks/items |
know what needs to be done and by when |
|
user |
prioritize tasks/items |
efficiently get tasks/items done on time |
|
user |
hide private contact details by default |
minimize chance of someone else seeing them by accident |
|
user |
import students/people from a text file |
it is easier to enter large numbers of people |
|
user |
add profile pictures |
know who the people I am working with are |
|
user |
mass email students/people based on a tag |
easily email/inform a class of students of announcements |
|
user |
set reminders for certain events |
have an email sent to me before the actual event so that I don’t forget |
|
user with many persons in the address book |
combine groups/tags |
better work with people who have similar interests/work |
Appendix C: Use Cases
(For all use cases below, the System is Your TA
and the Actor is the user
, unless specified otherwise)
Use case: Delete person
MSS
-
User requests to list persons.
-
Your TA shows a list of persons.
-
User requests to delete a specific student in the list.
-
Your TA deletes the student and all related entries (if any).
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Your TA shows an error message.
Use case resumes at step 2.
-
Use case: Updating the display picture
MSS
-
User requests to update the display picture of a person with an image file filepath.
-
Your TA shows a list of persons that match the search query.
-
Your TA reads the image file, duplicates it and updates the display picture of the person.
Use case ends.
Extensions
-
3a. The filepath provided does not lead to any valid image file.
-
3a1. Your TA shows an error message.
Use case ends.
-
Use case: Find a person by name
MSS
-
User requests to find a person by name.
-
Your TA shows a list of persons that match the search query.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
1.8.0_60
or higher installed. -
Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Should be intuitive or easily understood after reading the User Guide.
-
Should not exceed the size of 100 MB.
-
Should respond to any requests within 3 seconds.
-
Should not modify and copy any user’s personal data on the computer.
-
A user’s data should be password protected.
Appendix E: Glossary
- Collision resistance
-
Two different inputs to a function will very unlikely provide the same output.
- Deadline
-
The date for which certain tasks are due to be done.
- Feature
-
A specific function of the program.
- Import
-
Bring into the application from an external source.
- Integer overflow
-
When the value of the integer exceeds the maximum possible value value that can be stored.
- Logic
-
The set of commands that can be executed by the application.
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- Model
-
The internal memory used when application is running.
- Parser
-
A converting function or class that takes in raw input and separates it into its usable components for other methods.
- Private contact detail
-
A contact detail that is not meant to be shared with others.
- SHA-256
-
A cryptographic hash that is akin to a 'signature' for a text or a data file. SHA-256 generates an almost-unique 256-bit (32-byte) signature for a text.
- Storage
-
The set of instructions to store specific states and data of the application when application is not running so that it can be loaded back into the application when application is started again.
- Tags
-
Keywords tied to categories or people.
- Tasks
-
A command to be executed.
- To-do list
-
A list of things to do.
- User interface
-
The visible interface that the user will be seeing when using the application.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
F.1. Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
-
F.2. Deleting a person
-
Deleting a person while all persons are listed
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list.-
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No person is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size)
Expected: Similar to previous.
-
-
The corresponding display picture image to the person deleted is expected to be deleted upon next application start up and log in (to same account).
F.3. Adding participation marks for a person
-
Adding participation marks to a person while all persons are listed.
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list.-
Test case:
markPart 1 marks/50
Expected: First person is updated with the addition of the marks. Success message shown in the status message. Timestamp in the status bar is updated. -
Test case:
markPart 0 marks/50
Expected: No marks are added to anyone. Error details shown in the status message. Status bar remains the same. -
Test case:
markPart 1
Expected: No marks are added to anyone. Error details shown in the status message. Status bar remains the same. -
Other incorrect markPart commands to try:
markPart
,markPat x marks/50
(where x is larger than the list size)
Expected: Similar to previous.
-
-
-
Adding participation marks to a person when you searched for a person using
find
-
Prerequisites: Find a person using the
find
command. At least one person in the list returned.-
Test case:
markPart 1 marks/50
Expected: First person is updated with the addition of the marks. Success message shown in the status message. Timestamp in the status bar is updated. List is still filtered. -
Test case:
markPart 0 marks/50
Expected: No marks are added to anyone. Error details shown in the status message. Status bar remains the same. List is still filtered. -
Test case:
markPart 1
Expected: No marks are added to anyone. Error details shown in the status message. Status bar remains the same. List is still filtered.
-
-
F.4. Adding/Updating display picture for a person
-
Adding display picture when adding a new (non-duplicate) person while all persons are listed.
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. Not adding a duplicate person.-
Assumptions: There is a valid image file at the specified location.
-
Test case:
add n/John Doe m/A1234567J p/98765432 e/johnd@example.com a/COM1 B#1-04 dp/C:\Users\Hello\Desktop\picture.jpg
Expected: John Doe is added with the specified details and image. Success message shown in the status message. Timestamp in the status bar is updated. -
Test case:
add n/John Doe m/A1234567J p/98765432 e/johnd@example.com a/COM1 B#1-04 dp/C:\Users\Hello\Desktop\picture.jpg
Expected: John Doe is added with the specified details and has the default display picture. Success message shown in the status message. Timestamp in the status bar is updated.
-
-
-
Adding display picture to an already existing person while all persons are listed.
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list.-
Assumptions: There is a valid image file at the specified location.
-
Test case:
updateDP 1 dp/C:\Users\Hello\Desktop\picture.jpg
Expected: First person’s display picture is updated with the image. Success message shown in the status message. Timestamp in the status bar is updated. -
Test case:
updateDP 1 dp/
Expected: First person’s display picture is updated to the default display picture. Success message shown in the status message. Timestamp in the status bar is updated.
-
-
F.5. Saving and loading of the image files
-
Deleting image files while the application is still running.
-
Prerequisites: There exists some persons already added and are utilizing a display picture.
-
Test case: Delete all images from the
data/displayPic
folder.
Expected: Upon next refresh of the list that causes changes, the affected persons will be reset back to the default profile picture.
-
-
-
Checking proper deletion of image files
-
Prerequisites: None of the existing persons in Your TA have a display picture.
-
Test case:
-
Adding multiple images using the
updateDP
oredit
command. E.g executingupdateDP 1 dp/C:\Users\Hello\Desktop\picture.jpg
twice. -
Open the
data/displayPic
folder to note that there are 2 image files created. -
Close and restart the application and log back in to the same account.
Expected: Unused image files will be deleted, only one image file remains.
-
-
-
-
Deleting image files while the application is not running.
-
Prerequisites: There exists some persons already added and are utilizing a display picture.
-
Test case: Delete all images from the
data/displayPic
folder.
Expected: Upon loading up the application, the affected persons will be reset back to the default profile picture.-
Adding a task while all tasks are listed.
-
-
-
F.6. Adding a Task
-
Prerequisites: List all tasks using the
listTask
command. Multiple tasks in the list.-
Test case:
addTask title/meeting desc/there is team meeting for CS2103 by/20-04-2018 priority/3
Expected: a task is added with title, description, deadline and priority stated in the command. Details of the added task shown in the status message. Timestamp in the status bar is updated. -
Test case: 'addTask title/meeting` Expected: No task is added. Error details shown in the status message. Status bar remains the same.
-
Other incorrect addTask commands to try:
addTask title/meeting priority/1
,addTask by/20-04-2018 priority/3
Expected: similar to previous.-
Adding a task while current month tasks are listed.
-
-
-
Prerequisites: List all current month tasks using the
listCurrentTask
command. Multiple tasks in the list.-
Test case:
addTask title/meeting desc/there is team meeting for CS2103 by/20-04-2018 priority/3
Expected: a task is added with title, description, deadline and priority stated in the command. All tasks again are shown in the list. Details of the added task shown in the status message. Timestamp in the status bar is updated. -
Test case: 'addTask title/meeting` Expected: No task is added. Error details shown in the status message. Status bar remains the same.
-
Other incorrect addTask commands to try:
addTask title/meeting priority/1
,addTask by/20-04-2018 priority/3
Expected: similar to previous.
-
F.7. Deleting a Task
-
Deleting a task while all tasks are listed.
-
Prerequisites: List all tasks using the
listTask
command. Multiple tasks in the list.-
Test case:
deleteTask 1
Expected: First task is deleted from the list. Details of the deleted task shown in the status message. Timestamp in the status bar is updated. -
Test case:
deleteTask 0
Expected: No task is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect deleteTask commands to try:
deleteTask
,deleteTask x
(where x is larger than the list size) Expected: similar to previous.
-
-
F.8. Editing a Task
-
Editing a task while all tasks are listed.
-
Prerequisites: List all tasks using the
listTask
command. Multiple tasks in the list.-
Test case:
editTask 1 title/meeting priority/3
Expected: First task is edited with title meeting and priority 3. It is shown last in the list. Details of the edited task shown in the status message. Timestamp in the status bar is updated. -
Test case: 'editTask 1` Expected: No task is edited. Error details shown in the status message. Status bar remains the same.
-
Other incorrect addTask commands to try:
editTask title/meeting priority/1
,editTask x by/20-04-2018 priority/3
(where x is larger than the list size) Expected: similar to previous.
-
-